티스토리 뷰
1. canvas 요소 및 ref 정의
//html
<canvas ref={canvasRef} />
//typescript
const canvasRef = useRef<HTMLCanvasElement>(null);
//rust
//dom에 직접 접근하지 않음.
//wasm 사용 중이므로 함수의 인자로 넘겨주는 방식
fn canvas(canvas: web_sys::HtmlCanvasElement) { ... }
2. '2d' context 정의
- context는 화면에 그림을 그릴 펜이라고 생각하면 된다.
//typescript
//ref가 null일수 있으므로 옵셔널 체이닝 정의
let context = canvasRef.current?.getContext('2d');
//rust
let context = canvas
.get_context("2d")
.unwrap()
.unwrap()
.dyn_into::<web_sys::CanvasRenderingContext2d>()
.unwrap();
3. '2d' context method
//typescript
context.beginPath() => 새로운 경로 생성
context.arc() => 원을 그리는 메서드(너비, 높이, 반경, 시작지점, 종료지점, 반대/정방향);
context.clearRect() => 특정 부분을 지우는 직사각형
context.fill() => 경로의 내부를 채워서 내부가 채워진 도형을 그림
context.fillText(문자열, x좌표, y좌표) => 주어진 위치에 text를 채움
context.lineTo(x좌표, y좌표) => 직선
context.moveTo(x좌표, y좌표) => 지정된 좌표로 도형을 이동
context.stroke() => 도형을 출력
//rust
context.begin_path()
context.arc().unwrap(); => 원 그림
context.move_to().unwrap(); => context를 이동시킴
context.stroke() => 그린것 출력
wasm으로 canvas에 그림
use std::f64;
use wasm_bindgen::JsCast;
pub fn canvas_base(canvas: web_sys::HtmlCanvasElement) {
let context = canvas
.get_context("2d")
.unwrap()
.unwrap()
.dyn_into::<web_sys::CanvasRenderingContext2d>()
.unwrap();
context.begin_path();
let width: u32 = canvas.width();
let height: u32 = canvas.height();
let margin: f64 = 105.0;
let radius = (width / 2) as f64 - margin;
let draw_cicle = || {
context
.arc((width / 2) as f64, (height / 2) as f64, radius, 0.0, f64::consts::PI * 2.0)
.unwrap();
};
let calculation = || {
context.move_to(width as f64 / 2.0 + 36.0, height as f64 / 2.0);
context.arc(width as f64 / 2.0, height as f64 / 2.0, width as f64 / 8.5, 0.0, f64::consts::PI).unwrap();
context.move_to(width as f64 / 2.0 + 20.0, height as f64 / 2.5);
context
.arc(width as f64 / 2.0 + 15.0, height as f64 / 2.5, 5.0, 0.0, f64::consts::PI * 2.0)
.unwrap();
context.move_to(width as f64 / 2.2 + 5.0, height as f64 / 2.5);
context
.arc(width as f64 / 2.2, height as f64 / 2.5, 5.0, 0.0, f64::consts::PI * 2.0)
.unwrap();
};
fn draw(context: &web_sys::CanvasRenderingContext2d){
context.stroke();
}
draw_cicle();
calculation();
draw(&context);
}
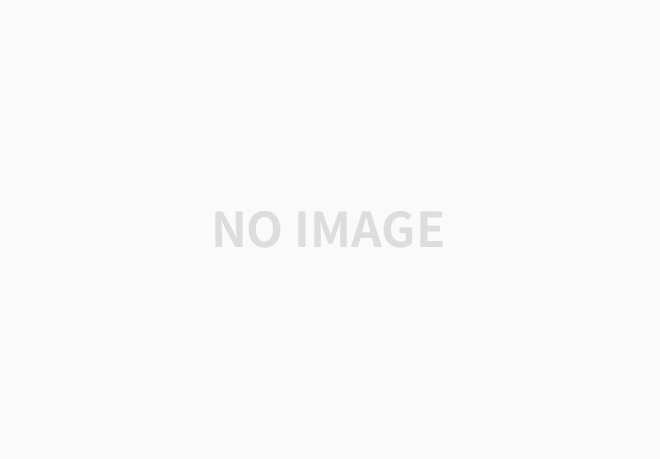
'front-end > HTML5 canvas' 카테고리의 다른 글
HTML Canvas 최적화 (0) | 2023.05.03 |
---|